C++之类型转换函数
一、转换构造函数的学习:
1、回忆数据类型转换:
在平时写代码的时候,最怕的就是那种隐式数据类型转换了,一不小心,软件就bug不断;而显示数据类型(一般是程序自己去强制类型转换,这个是我们能够明显的识别和掌控的)。为此我们这里总结了一副隐式类型转换的图:
下面我们来几个隐式转换的例子:
代码版本一:
#include <iostream>
#include <string>
int main()
{
short s ='a';
unsigned int ui = 100;
int i = -200;
double d = i;
std::cout<<"d =" << d <<std::endl;
std::cout<<"ui= "<<ui<<std::endl;
if((ui+i)>0)
{
std::cout<<"Postive"<<std::endl;
}
else
{
std::cout<<"Negative"<<std::endl;
}
return 0;
}
输出结果:
root@txp-virtual-machine:/home/txp# ./a.out
d =-200
ui= 100
Postive
注解:这里我们明显发现(-200+100)还是大于0,这显然不符合正常人的思维了;所以我们仔细分析一下,发现这里肯定是进行了隐式转换了,为此我们再加一条语句看看(ui+i)的值到底是多少:
代码版本二:
#include <iostream>
#include <string>
int main()
{
short s ='a';
unsigned int ui = 100;
int i = -200;
double d = i;
std::cout<<"d =" << d <<std::endl;
std::cout<<"ui= "<<ui<<std::endl;
if((ui+i)>0)
{
std::cout<<"(ui+i) = "<<ui+i<<std::endl;
std::cout<<"Postive"<<std::endl;
}
else
{
std::cout<<"Negative"<<std::endl;
}
return 0;
}
输出结果:
root@txp-virtual-machine:/home/txp# ./a.out
d =-200
ui= 100
(ui+i) = 4294967196
Postive
注解:通过打印(ui+i)的值我们发现,i原本是int数据类型,这里隐式转换成无符号的数据类型了
为了让大家更加理解隐式的转换,我们下面再来一个例子:
代码版本三:
#include <iostream>
#include <string>
int main()
{
short s ='a';
unsigned int ui = 100;
int i = -200;
double d = i;
std::cout<<"d =" << d <<std::endl;
std::cout<<"ui= "<<ui<<std::endl;
if((ui+i)>0)
{
std::cout<<"(ui+i) = "<<ui+i<<std::endl;
std::cout<<"Postive"<<std::endl;
}
else
{
std::cout<<"Negative"<<std::endl;
}
std::cout<<"sizeof(s+'b') = "<<sizeof(s+'b')<<std::endl;
return 0;
}
输出结果:
root@txp-virtual-machine:/home/txp# g++ test.cpp
root@txp-virtual-machine:/home/txp# ./a.out
d =-200
ui= 100
(ui+i) = 4294967196
Postive
sizeof(s+'b') = 4
注解:这里我们发现sizeof出来的内存大小是4个字节大小;其实这里编译器把short和char类型的都转换int类型了,所以最终两个int数据相加,所占的内存大小就是int类型了。
所以咋们平时在写代码的时候,脑袋里面要有这种写代码谨慎的思维,防止出现这种隐式转换的情况出现,养成写代码的好习惯
2、普通类型与类类型之间能否进行类型转换,类类型之间又是否能够类型转换呢?
为了说明这些问题,咋们通过实际的代码测试来看看啥情况:
代码:普通类型转换成类类型
#include <iostream>
#include <string>
class Test{
public:
Test()
{
}
Test(int i)
{
}
};
int main()
{
Test t;
t =6; 从 C 语言角度,这里将 5 强制类型转换到 Test 类型,只不过编译器 在这里做了隐式类型转换
return 0;
}
输出结果(显示可以编译通过)
root@txp-virtual-machine:/home/txp# g++ test.cpp
root@txp-virtual-machine:/home/txp# ./a.out
代码类类型转换为普通类型
#include <iostream>
#include <string>
class Test{
public:
Test()
{
}
Test(int i)
{
}
};
int main()
{
Test t;
int i = t;
return 0;
}
输出结果(没有编译通过)
root@txp-virtual-machine:/home/txp# g++ test.cpp
test.cpp: In function ‘int main()’:
test.cpp:21:14: error: cannot convert ‘Test’ to ‘int’ in initialization
int i = t;
^
代码类类型与类类型之间的转换:
#include <iostream>
#include <string>
class Value{
};
class Test{
public:
Test()
{
}
Test(int i)
{
}
};
int main()
{
Test t;
Value i;
t=i;
return 0;
}
输出结果(暂时还是不行,编译不通过):
root@txp-virtual-machine:/home/txp# g++ test.cpp
test.cpp: In function ‘int main()’:
test.cpp:27:7: error: no match for ‘operator=’ (operand types are ‘Test’ and ‘Value’)
t=i;
^
test.cpp:27:7: note: candidate is:
test.cpp:9:7: note: Test& Test::operator=(const Test&)
class Test{
^
test.cpp:9:7: note: no known conversion for argument 1 from ‘Value’ to ‘const Test&’
说明:上面的例子,我们只是简单的按照实际角度出发,发现确实有写转换行不通。那么真理到底是怎样的?我们接着往下看
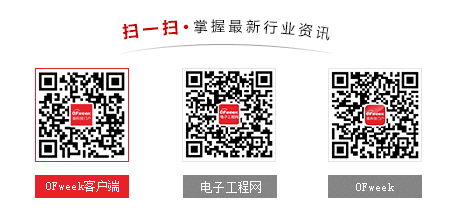
图片新闻
技术文库
最新活动更多
-
即日-12.26立即报名>>> 【在线会议】村田用于AR/VR设计开发解决方案
-
1月8日火热报名中>> Allegro助力汽车电气化和底盘解决方案优化在线研讨会
-
1月9日立即预约>>> 【直播】ADI电能计量方案:新一代直流表、EV充电器和S级电能表
-
即日-1.14火热报名中>> OFweek2025中国智造CIO在线峰会
-
即日-1.20限时下载>>> 爱德克(IDEC)设备及工业现场安全解决方案
-
即日-1.24立即参与>>> 【限时免费】安森美:Treo 平台带来出色的精密模拟
推荐专题
发表评论
请输入评论内容...
请输入评论/评论长度6~500个字
暂无评论
暂无评论